At Cylynx, we build tools and solutions to help financial crime investigators connect the dots between their data points. This calls for a custom graph application to provide additional time series and investigative functionalities. Building a connector to Neo4j was one of our most requested features with its popularity within the financial services space. In this post, we document how we used use-neo4j hooks to quickly create a connector with Motif, our graph investigation software.
Here’s a demo of the final integration:
What Is Motif?
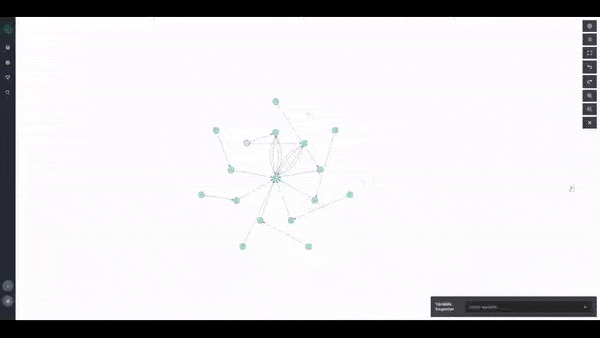
Motif is a graph visualization and investigation application built on top of React, D3 and G6. It makes analyzing financial transactions easy with multiple layout options, filtering panels and edge bundling. Currently, it supports importing data as JSON files, edge-list CSVs, node-edge CSVs and from a Neo4j database directly.
As it is built on top of React, it is easy to customize and add in new data connectors. We use G6 and its React binding library Graphin as they contain many out of the box styling and layout options. The libraries require a graph data input to be specified as a node and edge JSON file, similar to D3, visjs and many other popular javascript graphing libraries.
What Is use-neo4j Hooks?
From its Github repository:
A set of components and hooks for building React applications that communicate to Neo4j. This is a package intended to speed up the development by reducing the amount of boilerplate code required.
Since we were already building a React application, the library made it very easy to speed up the development process and create an integration with Neo4j.
Hooking Up
To create a good user experience, we wanted to separate the database connection step from the querying step. A user can just connect Motif to their desired database for the first time and can then execute multiple queries without needing to re-authenticate.
The pseudo-code to achieve this is outlined below:
use-neo4j hooks provides a set of functions and React hooks to manage the database connections and querying process. We use them in the ConnectDatabase and ExecuteQuery components. In the parent connector, we keep track of the driver settings and the step which the user is on.
Connect to Database Component
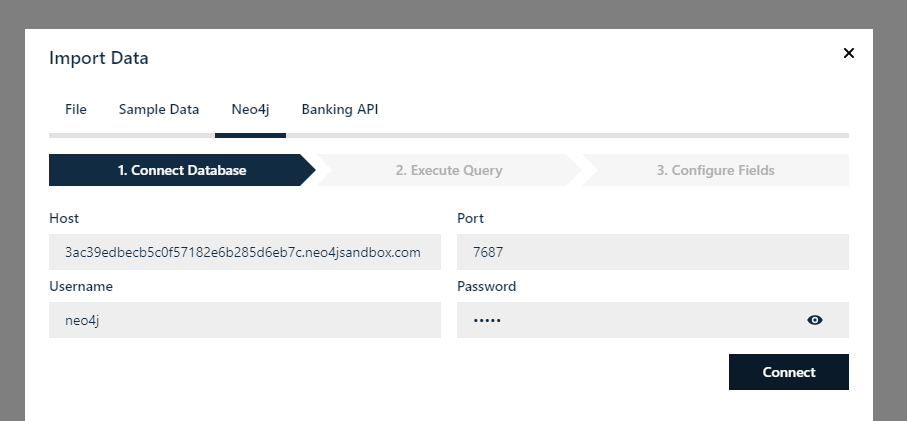
The ConnectDatabase component is responsible for initializing the driver and we use the createDriver function to set up a bolt connection with Neo4j.
While use-neo4j hooks comes with a built-in login form, we want to have greater control of the styling and use our own form component for that to allow the user to key in her database connection details (host, port, username & password).
On submission of the form, we create a driver and tests its connection. If successful, we store that driver instance in the state of our parent component. Otherwise, we display an error message.
Query Component
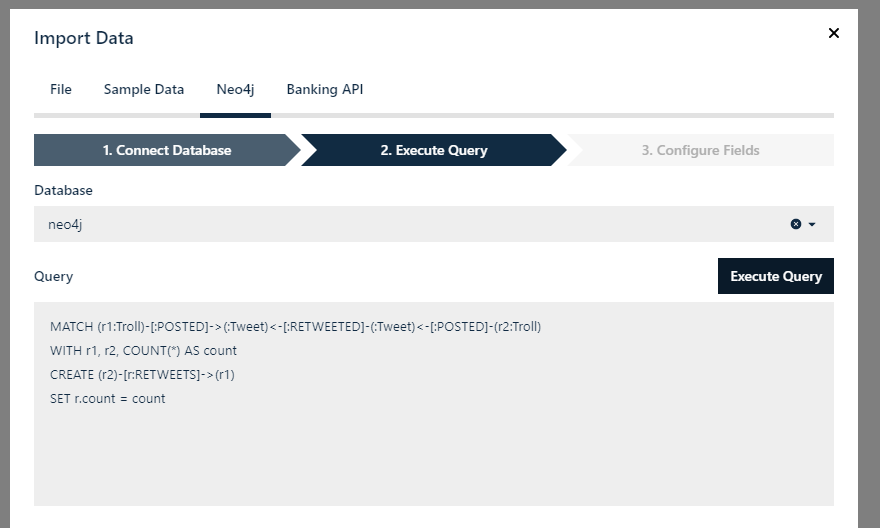
The ExecuteQuery component contains the Neo4jProvider which manages the driver state and a CypherQuery component which is a text form that allows a user to key in her query and submits it to the database.
We also use the useDatabase hook to populate a list of database options that the user can choose from.
From Neo4j to D3 Format
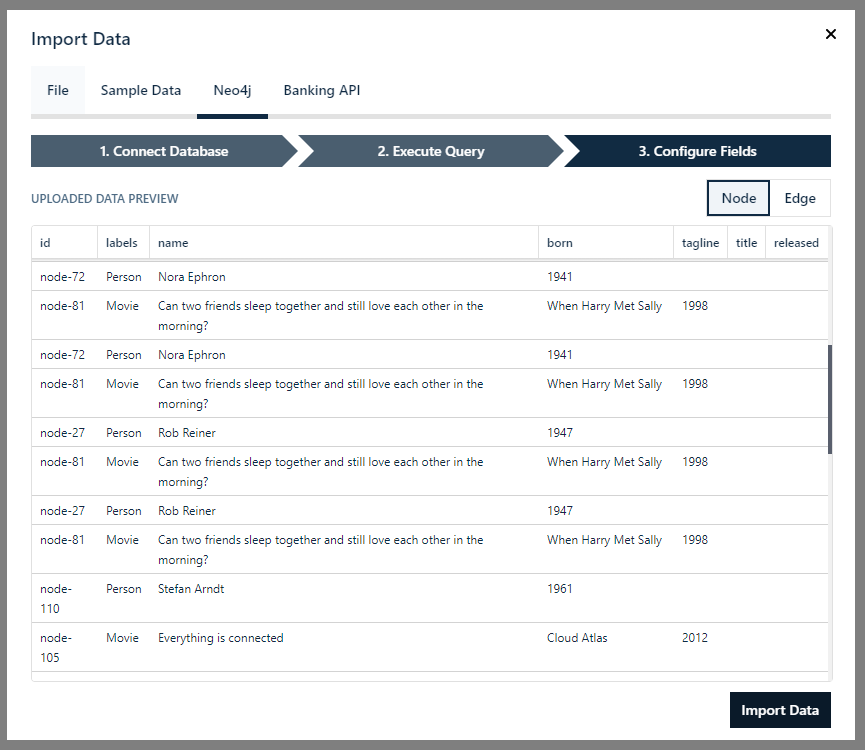
Next, we have to convert the returned results from Neo4j which is a list of records into a format that is D3 compatible. neovis.js provides a very good guide on how to achieve this and for the most part, we copied their code.
Here’s the full code for reference (you might need to adapt the types on your application)
Developer Tips
- Use the Neo4j sandbox to speed up the development process. The one-click setup and easy access to multiple dataset helps us speed up the testing process
- Neo4j records data format supports multiple labels. For our application, we just take the first label. Another alternative would be to spread them out in the returned array.
- use-neo4j hooks helps save time if you are already working on a React application and looking to quickly build an integration with Neo4j.
Creating a Neo4j React App with use-neo4j Hooks was originally published in Neo4j Developer Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.